14. Blog Design¶
Studios are in-class activities to give you hands-on practice with new concepts. The first half is the Walkthrough, an instructor-led programming problem. The second half is for you to work on individually or in pairs in class.
These problems are not graded, and you are not obligated to finish them. Get as far as you can while in class, and use them as an opportunity to play with and solidify new concepts.
Walkthrough¶
In this walkthrough, we’ll build a couple of classes using inheritance. We also introduce the idea of a class diagram, which is a method of visually representing classes and the way they relate to each other.
When viewing these class diagrams, there are 4 main components:
- Class name - the class name is at the top of the box representing a given class
- Methods - the methods that the class contains, along with the arguments that each method takes
- Fields - the fields/properties that the class contains (aka, attributes)
- Inheritance - an arrow from one class to another indicates that the former class inherits from or extends the latter class
These class diagrams are special cases of a more general format for representing classes and objects visually, known as Universal Modeling Languages (UML). You can read more about UML at Wikipedia.
The code we’ll write mimics the idea of a multi-format blog platform, such as Tumblr. We’ll model a blog post as a basic entity, but also include multiple different types of posts that have different behavior based on the media that they are presenting. For the walkthrough, we’ll build Post
and VideoPost
classes.
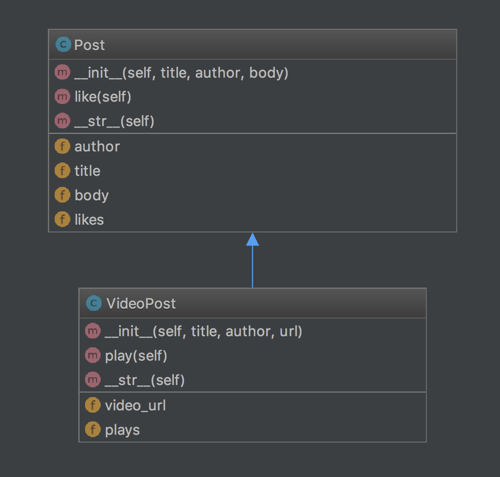
Note
In the walkthrough, we use the syntax Post.__init__(self, [args] )
to invoke the superclass’ constructor. This is totally valid, but isn’t the preferred way to do so. A better way is to use super().__init__( [args] )
. This is a better method because if your class has multiple parent classes – that is, it’s parent class also has a parent class, and so on – then each of the superclass constructors is invoked in order.
We use this less-preferred method because the version of Python used in this book doesn’t support the preferred method. When using Python in most situations, you’ll be able to use the preferred method.
Studio¶
Create two new classes, ImagePost
and LinkPost
, based on the class diagram below. The output of your code should match this sample output:
Little Scream - Love As a Weapon played 2 times
10 Best Albums of 2016 by Chris Bay
Cats in space by Crystal Martin
LaunchCode's LC101: https://www.launchcode.org/lc101
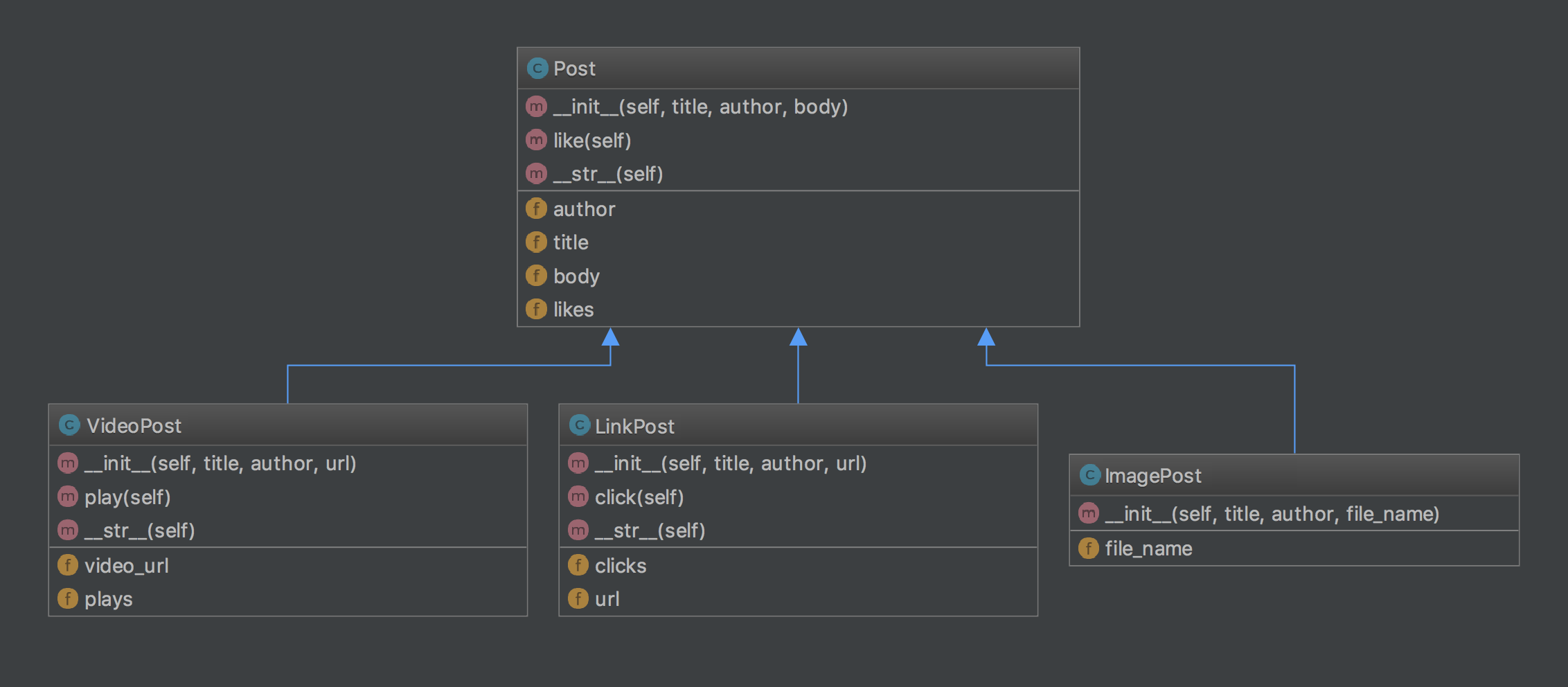